Hi! In my spare time from website development and SEO we are doing a fan version of Shining Force.
we are working on the concept and code of the game
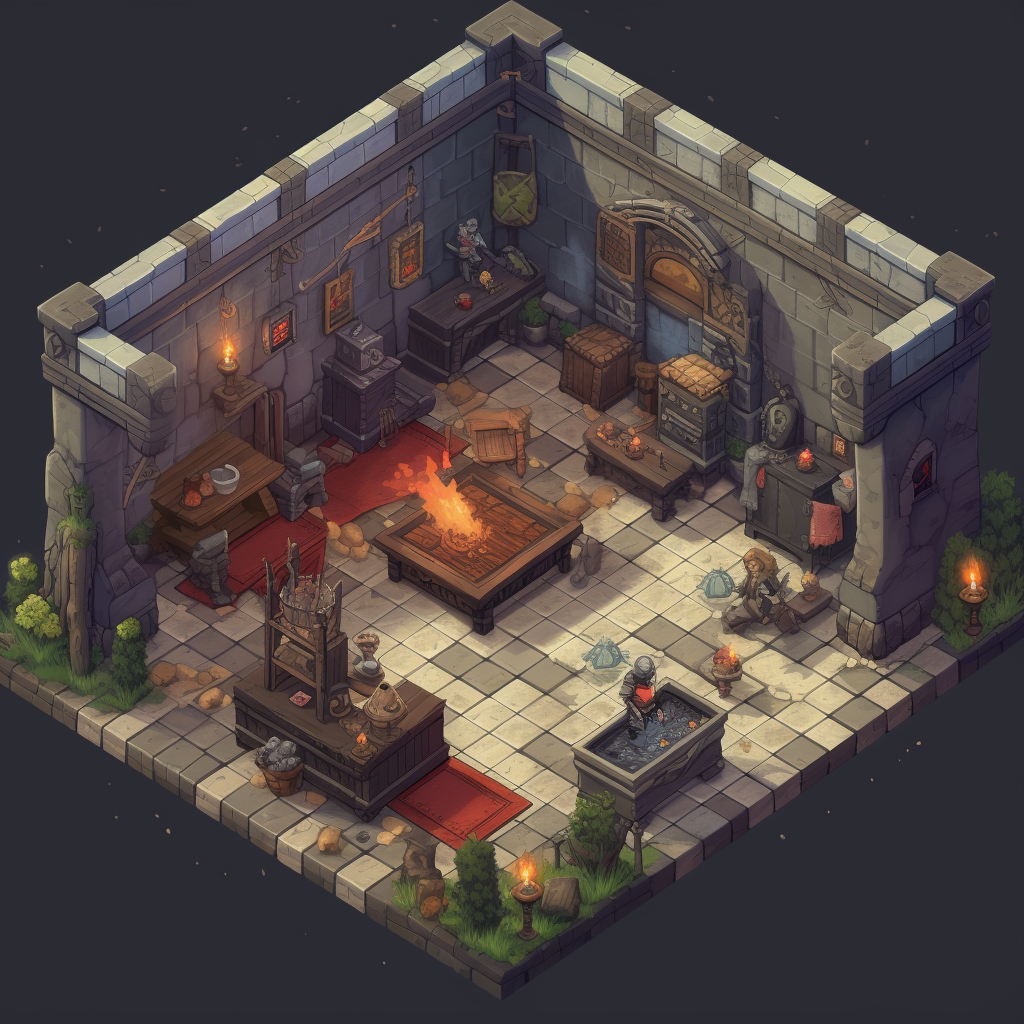
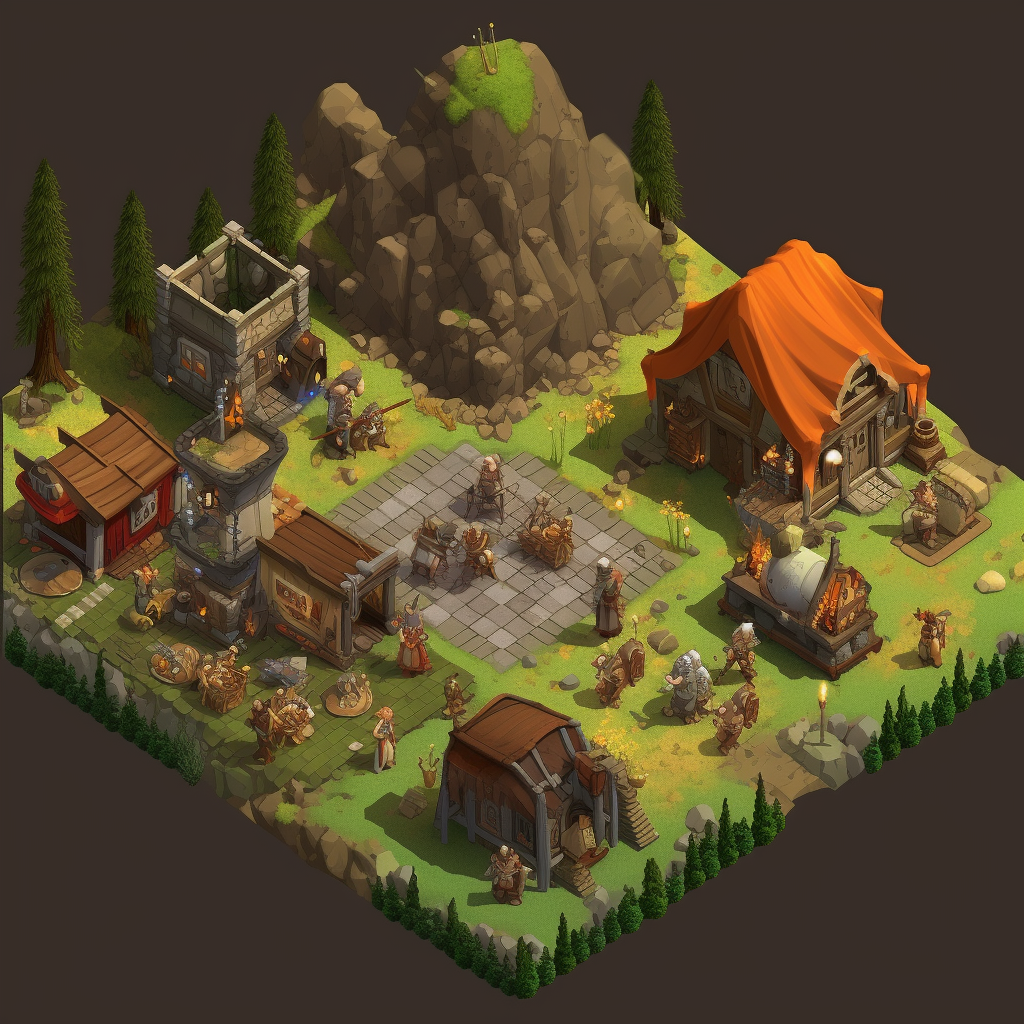
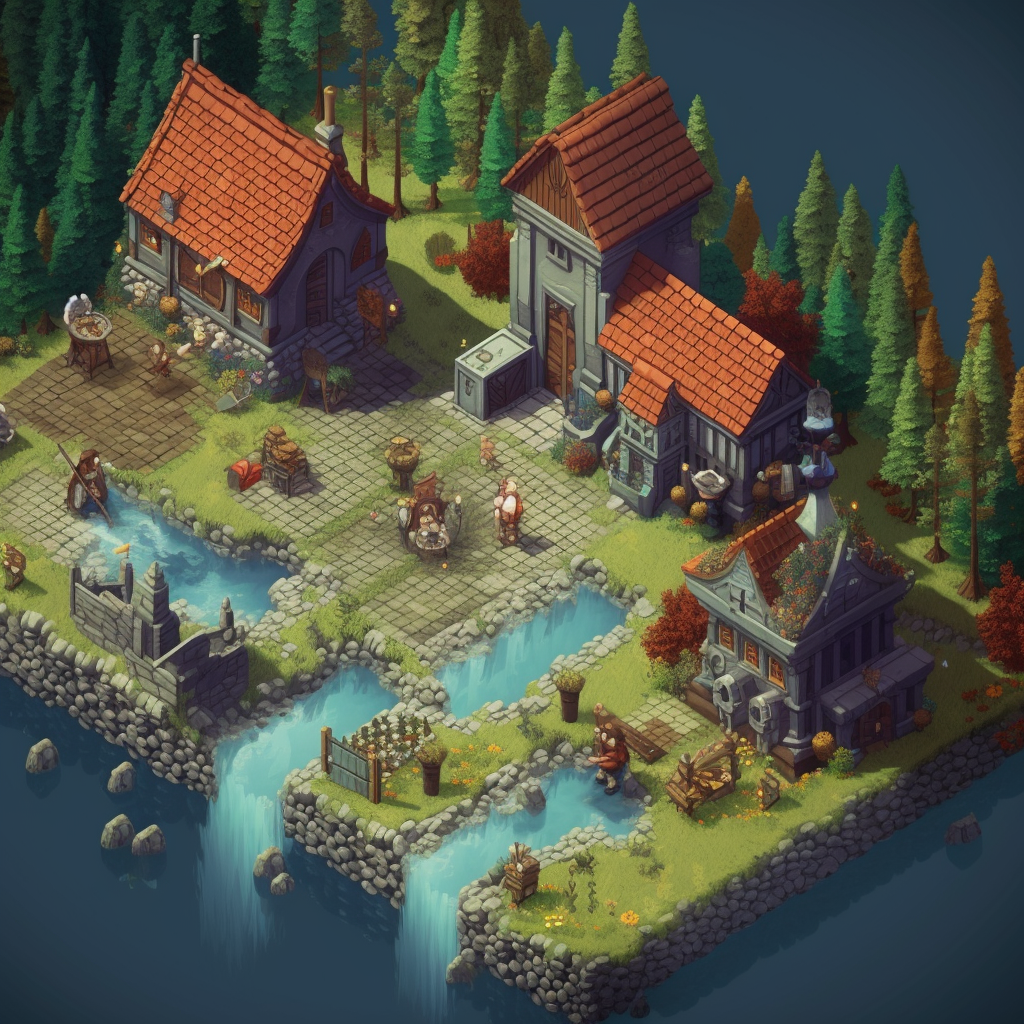
This code includes an Character class representing both the player and enemies. Each character has HP, MP, attack, defense, magic, agility, and level parameters. The player and enemies also have their own choose_action method for choosing what to do in battle, and the player has a use_magic method for casting spells.
The combat function takes two Character instances as parameters and simulates a turn-based battle between them. It prints out what happens in each turn and adjusts the HP and defense of characters as necessary. The combat function runs until one of the characters is defeated.
This code is just an example and can be extended to create a full game. You can add more characters, items, quests, and explore different battles scenarios.
The combat function takes two Character instances as parameters and simulates a turn-based battle between them. It prints out what happens in each turn and adjusts the HP and defense of characters as necessary. The combat function runs until one of the characters is defeated.
This code is just an example and can be extended to create a full game. You can add more characters, items, quests, and explore different battles scenarios.